Data Structures and Algorithms (DSA) form the backbone of modern computer science and programming techniques. They provide the tools to store, organize, and manipulate data efficiently while solving problems with optimized logic.
Mastering DSA is essential for building scalable applications and excelling in technical interviews. As one of the most comprehensive data structures and algorithms best tutorial resources, this guide provides the tools to store, organize, and manipulate data efficiently while solving problems with optimized logic.
Key Takeaways
- DSAs are the building blocks of efficient programming. They enable scalable and optimized solutions for complex problems.
- Mastering both linear (arrays, stacks, and linked lists) and non-linear (trees and graphs) data structures equips developers to handle diverse scenarios.
- Techniques like divide-and-conquer, dynamic programming, and greedy algorithms provide frameworks for solving problems efficiently.
- DSA is widely used in real-world systems, including navigation tools, recommendation engines, and social networks, showcasing its importance in modern computing.
- Innovations like quantum algorithms, AI-driven data structures, and blockchain optimizations are pushing DSA into new frontiers.
What Are Data Structures?
Data structures represent specialized ways to store data and organize elements in computer memory. They can be divided into two main categories: linear and non-linear.
Linear Data Structures
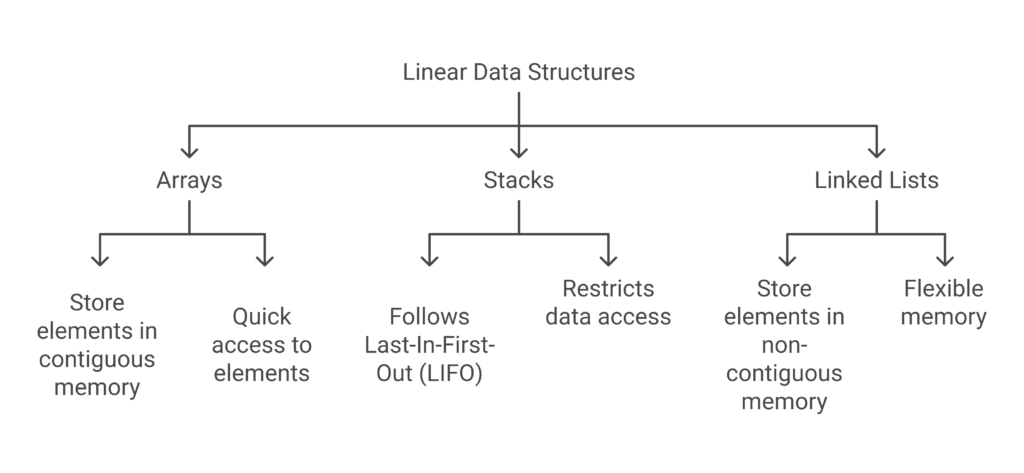
Code examples: https://onecompiler.com/c/434j4wqjg
Linear data structures store elements sequentially. The most fundamental linear data structures include arrays, stacks and linked lists.
1. Arrays
Arrays store elements in contiguous memory locations, making them ideal for situations requiring quick access to elements. Each element is accessed using its index, which ensures efficient read/write operations. However, arrays have a fixed size, which can limit their flexibility.
2. Stack
Stacks follow Last-In-First-Out (LIFO) principles. Unlike arrays, stacks restrict data access to maintain data integrity, allowing insertion and deletion only at the top. This structure is widely used for function calls, expression evaluation, and undo operations in programs.
3. Linked Lists
Linked lists store elements in non-contiguous memory locations, with each node containing data and a pointer to the next node. This data structure proves especially useful when memory allocation needs flexibility, as nodes can be dynamically added or removed without reallocating the entire list.
Non-Linear Data Structure Types
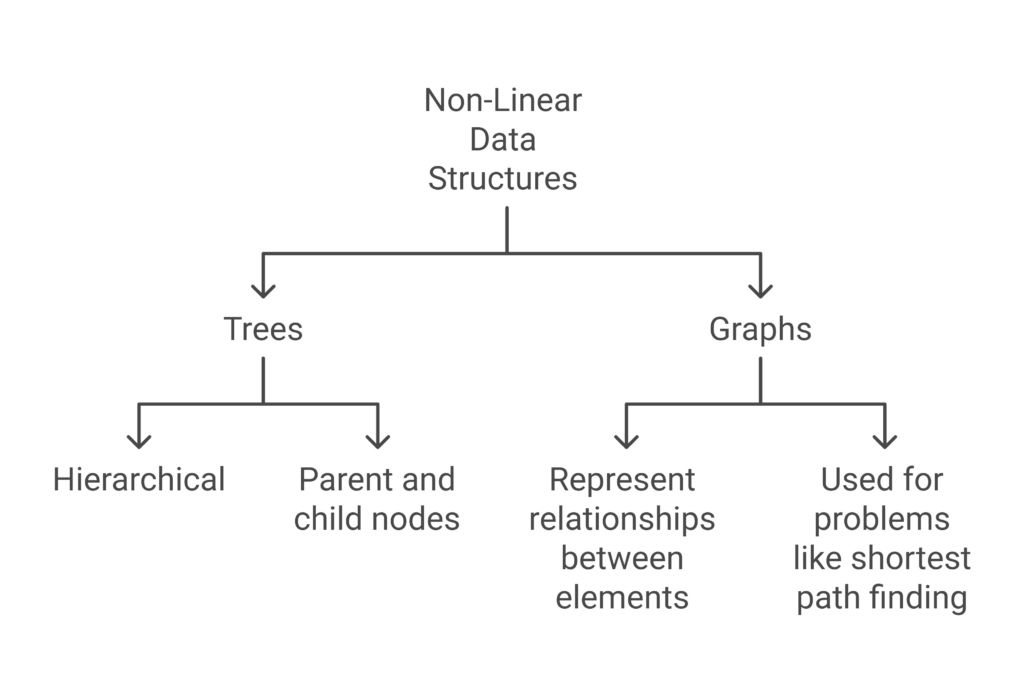
Code examples: https://onecompiler.com/c/434j5gswx
Non-linear data structures organize elements hierarchically or in networks. These complex data structures include:
1. Tree Data Structure
Trees store data in hierarchical relationships with parent and child nodes. The root node is the topmost element, and each node can have zero or more child nodes. Binary trees are used for searching, sorting, and representing hierarchical data like file systems.
2. Graph Data Structures
Graphs represent relationships between elements using vertices (nodes) and edges (connections). They are crucial for solving problems like finding the shortest path, analyzing social networks, and modeling real-world networks like roads and communication systems.
What Are Algorithms?
An algorithm is a systematic procedure for solving problems in a finite number of steps. Think of algorithms as detailed recipes that tell a computer exactly how to solve a known problem. Every time you use a navigation app to find the shortest path or search for a friend on social networks, you’re benefiting from sophisticated algorithms working behind the scenes.
Types of Algorithms
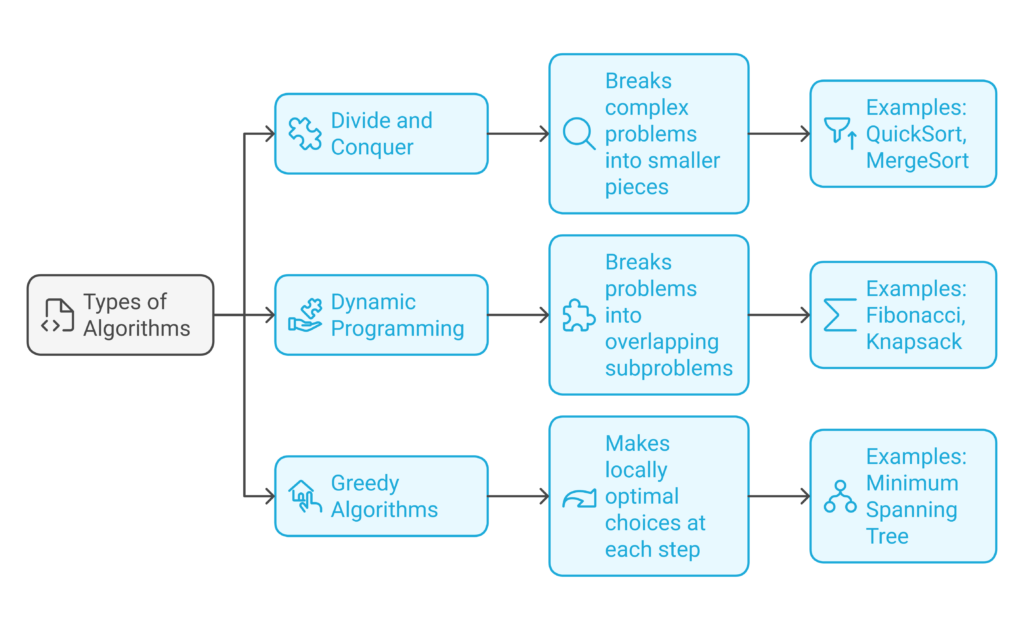
Code examples: https://onecompiler.com/c/434j5pd8v
1. Divide and Conquer Algorithms
These algorithms break complex problems into smaller, manageable pieces, solve each piece independently, and combine their solutions. Due to their recursive nature, they are efficient for tasks like sorting and searching. Examples include QuickSort and MergeSort.
2. Dynamic Programming
Dynamic programming solves problems by storing the results of subproblems to avoid redundant calculations. It is commonly used in optimization problems where solutions build upon previously solved subproblems. Examples include the Fibonacci sequence and the Knapsack problem.
3. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step, aiming to find the global optimum. They are efficient for problems where local choices lead to an optimal solution. Examples include finding the minimum spanning tree and the coin change problem.
Why You Should Learn DSA?
Learning algorithms and data structures provide several crucial benefits for software developers:
- Understanding DSA helps you break down complex problems into manageable pieces, a crucial skill in day-to-day programming.
- Knowledge of DSA helps write efficient code that performs well even with large inputs.
- Most software developer positions require strong DSA knowledge for problem-solving interviews.
You can find more tips on passing a coding interview in this comprehensive course “How to Pass a Coding Interview” by Edaqa Mortoray.
How to Start Learning Data Structures & Algorithms (DSA)?
For those beginning their journey with DSA, here is a simple approach to master the concepts.
Step 1: Build Strong Programming Fundamentals
Start your DSA journey by selecting a suitable programming language (Python, Java, or C++) and thoroughly understanding its basic syntax, control structures (loops, conditionals, functions), and object-oriented programming concepts (classes, inheritance, polymorphism). This foundation is crucial as it forms the basis for implementing complex data structures and algorithms later in your learning journey.
Step 2: Learn Basic Data Structures
Begin with fundamental data structures like
- Arrays (static and dynamic),
- Strings (pattern matching, manipulation),
- Linked lists (singly, doubly),
- Stacks (LIFO operations),
- Queues (FIFO operations),
- Basic tree structures (binary trees, BST).
Understanding these structures, its operations, and time complexities will help you solve complex problems efficiently and choose the right data structure for specific scenarios.
Step 3: Progress to Algorithms
When studying algorithm DSA concepts, it’s helpful to reference a data structures and algorithms list to track your progress. Many developers find that working through data structures and algorithm examples helps solidify their understanding. Whether you’re focusing on basic DSA and algorithms or advanced concepts, systematic practice is key to mastery.
- Sorting algorithms
- Searching algorithms
- Basic recursive algorithms
- Graph algorithms
This Beginner’s Guide to Data Structures & Algorithms by Cory Althoff is a great place to start your journey if you are new to data structures and algorithms.
Applications of DSA
Let’s consider several examples to better understand data structure algorithm applications in real-world scenarios.
1. Google Maps
- Uses graph data structures to represent road networks.
- Implements shortest-path algorithms for navigation.
- Optimizes route calculations using efficient data structures.
2. Social Media Platforms
- Uses graph databases for storing user connections.
- Uses recommendation algorithms for content suggestion.
- Implement efficient searching and sorting for user interactions.
3. Spotify
- Graph-based algorithms to analyze user listening patterns.
- Clustering algorithms to group similar songs and users.
- Custom data structures for storing and quickly accessing musical features.
- Efficient caching mechanisms to handle millions of weekly updates.
Common Challenges and Solutions
Learning Data Structures and Algorithms (DSA) can be daunting due to its abstract concepts and mathematical foundations. Here are some common challenges developers face and strategies to overcome them effectively:
1. Understanding Recursion
Recursion often poses difficulties because it requires thinking in terms of function calls and call stacks, which may not be intuitive initially. To master it:
- Start with visualization tools to trace recursive calls.
- Practice with simple problems like factorial calculation before moving to complex tree traversals.
- Use the “stack frame” mental model to understand recursive function calls.
- Implement both recursive and iterative solutions to understand trade-offs.
2. Mastering Time Complexity Analysis
Analyzing time complexity can be overwhelming, especially when dealing with nested loops and recursive functions. To overcome it:
- Begin with simple algorithms and their basic operations.
- Use visualization tools to understand how operation count grows with input size.
- Practice analyzing common algorithms before attempting complex ones.
- Focus on identifying dominant terms in complexity calculations.
3. Balancing Theory with Implementation
Many beginner developers struggle with translating theoretical concepts into working code. To overcome it:
- Focus on one concept at a time—start with arrays and lists before progressing to graphs and dynamic programming.
- Study sample codes to understand patterns and implementation techniques.
- Apply learned concepts in mini-projects like sorting visualizers, pathfinding simulators, or stack-based calculators.
Latest Trends and Research
The field of DSA continues to evolve with new technologies and requirements:
Quantum Algorithms
Recent developments in quantum computing have led to new algorithmic approaches:
- Quantum versions of classical algorithms like searching and sorting.
- Novel data structures optimized for quantum computers.
- Hybrid classical-quantum algorithms for specific problems.
AI-Driven Data Structures
Machine learning is revolutionizing traditional data structures:
- Self-adjusting data structures that adapt to usage patterns.
- Neural network-based index structures for faster searching.
- Learned data structures that optimize themselves based on data characteristics.
Blockchain Optimizations
The rise of blockchain technology has spawned new algorithmic challenges:
- Efficient consensus algorithms for distributed systems.
- Novel data structures for storing transaction histories.
- Optimized verification algorithms for smart contracts.
Conclusion
Mastering data structures and algorithms is an essential skill set for any software developer in modern computer programming. It provides the foundation for solving problems efficiently, writing optimized code, and excelling in technical interviews. Whether you’re building search engines, recommendation systems, or blockchain applications, DSA knowledge helps you confidently tackle any challenge.
FAQs on Data Structures & Algorithms (DSA)
How Can I Learn Data Structures Effectively?
Start with basic data structures and gradually progress to more complex implementations. Practice implementing various types of data structures regularly.
What Makes Algorithms Data Structures Important in Programming?
Algorithms provide systematic ways to solve problems efficiently, making them crucial for optimizing software systems and handling complex tasks.
How Do Data Structures Impact Program Performance?
Different data structures offer varying time complexity and space complexity characteristics, directly affecting program efficiency.
How Do I Decide Which Data Structure to Use for My Problem?
Choose a data structure based on:
- Access Patterns: Use arrays or lists for fast indexing and sequential access.
- Dynamic Data: Prefer linked lists for frequent insertions and deletions.
- Uniqueness: Use sets to avoid duplicate values.
- Key-Value Mappings: Opt for maps or dictionaries for quick lookups.
- Hierarchical Relationships: Trees are ideal for hierarchical data like file systems.
- Network Relationships: Graphs are best for modeling connections like social networks.
Which Programming Language Is Best for Learning DSA?
While any programming language works, choose one you’re comfortable with to focus on learning data structures and algorithm concepts.
How Are Data Structures Used in Real Life?
Data structures power everything from social networks to operating systems, making them fundamental to modern computing.
Are DSA Skills Still Relevant With Modern Tools Like AI and ML?
Yes, DSA remains highly relevant:
- Many machine learning techniques rely on sorting, searching, and optimization algorithms.
- Efficient data structures are crucial for managing massive datasets.
- Algorithms like hashing and trees form the core of these technologies.
What Is the Difference Between Iterative and Recursive Algorithms?
- Iterative Algorithms use loops for repetition and are generally easier to debug.
- Recursive algorithms call themselves repeatedly until a base condition is met, making them useful for problems like tree traversal but often requiring more memory due to stack usage.
How Important Is Time and Space Complexity Analysis?
Time and space complexity determine how efficiently an algorithm performs as input size grows.
- Time complexity measures the time required to complete an operation. For example, O(n) implies that performance grows linearly.
- Space complexity evaluates additional memory requirements. For instance, O(1) means constant memory use.
What Is Backtracking in Algorithms?
Backtracking is a technique used for solving problems recursively by trying out multiple solutions and discarding those that fail.